Developing a Robust Data Driven UI Using WPF - The Data Model
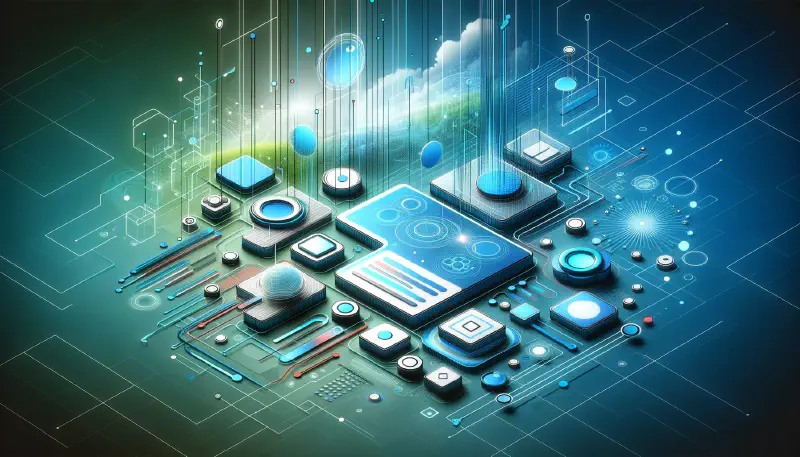
In the first post in the series I gave an overview of the pattern we’ll be using.
This post will go deeper into the DataModel, as defined in the previous post:
The DataModel is defined exactly as the Model in MVC; it is the data or business logic that stores the state and does processing of the problem domain.
The DataModel abstracts expensive operations such as data fetching without blocking the UI thread. It can keep data “alive” fetching it periodically from source (example: stock ticket), merge information from several sources etc. The DataModel is completely UI independent and pretty much straightforward to unit test.
The DataModel exposes data in a way that makes it easily consumable by WPF. As such, all if its public APIs, called by WPF for data-biding, must be called on the UI thread only. It must not block the UI thread because we want a robust functional UI so it usually performs operations on a background thread using the Dispatcher to send results back to the UI thread.
Therefore, the simplest DataModel implementation exposes several public Properties that expose data, implements INotifyPropertyChanged and/or INotifyCollectionChanged, and it abstracts the way information is fetched (using background threads to avoid blocking the UI thread when fetching the data is an expensive operation).
For two-way binding a commit and rollback mechanism, a dirty flag, etc. We’ll get to that later on…
As the DataModel implementation needs to abstract expensive data fetching operations and work with multiple threads we need some basic understanding of WPF’s threading model before we look at the DataModel implementation…
WPF Threading Model – A Quick Overview
A typical WPF uses two threads:
- Rendering thread – runs in the background and handles rendering
- UI thread – Receive inputs, handles events, paints the screen and runs application code.
The UI thread queues work items in a Dispatcher object. The Dispatcher object selects work items on a priority basis and runs each one to completion.
Every UI thread must have at least one Dispatcher, and each Dispatcher can only use one thread to execute work items.
Therefore, in order to build responsive UI that doesn’t block the UI thread, the application has to maximize the Dispatcher’s throughput by keeping work items small as to minimize the time the Dispatcher spends on processing them – which keeps other work items waiting causing the UI to lag.
In order to perform expensive operations without blocking the UI thread we can use a separate thread that will run in the background, leaving the UI thread free to process items in the Dispatcher queue. When the background thread is done processing it can report results back to the UI thread for display.
Doing this isn’t trivial as Windows only allows UI elements to be accessed by the thread that created them. This means that the background thread we used for some long-running task cannot access and update our UI when it is finished (or during work to show progress) – a background thread updating a control (such as a list box) during its rendering can cause strange UI behaviors that this limitation is there to prevent.
WPF uses the following design to enforce this kind of coordination between the UI thread and other threads:
Most of the classes in WPF derive from DispatcherObject. During construction, a DispatcherObject stores a reference to the Dispatcher linked with the current running thread – creating an association between itself and the thread that created it.
At the beginning of every method in the DispatcherObject, it calls VerifyAccess which compares the Dispatcher associated with the current thread with the Dispatcher stored during the object’s construction – if they do not match it throws an exception.
If only the creator of a DispatcherObject can access it, how can a background thread interact with the user?
The background thread does not access the UI directly but it can ask the UI thread to perform a task on its behalf by registering work items to its Dispatcher using it’s Invoke (for a synchronous call that returns when the UI thread finished executing the delegate) or BeginInvoke methods (which runs asynchronously)
The DataModel Class
So now, after the brief discussion on the use of the Dispatcher we can start coding our base DataModel class.
We’ll start with the simple class and constructor definition:
public abstract class DataModel : DispatcherObject, INotifyPropertyChanged
{
public DataModel()
{
}
We’re deriving from DispatcherObject because we need to have the Dispatcher available so that we can run background jobs that dispatch results to the UI thread.
As discussed earlier, each call to the DataModel should be made on the UI thread. Therefore we would like to enforce that limitation at the beginning of each publicly exposed API. The DispatcherObject class that we derived from contains a VerifyAccess() method that does just that. The method is public but unfortunately marked with the [EditorBrowsable(EditorBrowsableState.Never)] attributes which will make it hard to find for developers using driving their data model from our class.
To resolve this I simply defined a protected method as follows:
/// <summary>
/// Makes sure the call is in the correct thread (the UI thread) by comparing the current dispatcher
/// object with the dispatcher we got when the DataModel was created.
/// </summary>
[System.Diagnostics.Conditional("Debug")]
protected void VerifyCalledOnUIThread()
{
this.VerifyAccess();
}
This method will be visible to anyone deriving from our class and it simply calls VerifyAccess to make sure code is made from the UI thread.
The Conditional attribute makes this code execute only in debug bits avoiding this kind of assertion on retail bits – some performance gain.
In order to support asynchronous data fetching the DataModel should encapsulate the information about its state – valid (data fetched), invalid (error fetching data), fetching (processing).
public enum DataModelState
{
/// <summary>
/// The model is fetching data
/// </summary>
Fetching,
/// <summary>
/// The model is in an invalid state
/// </summary>
Invalid,
/// <summary>
/// The model has fetched its data
/// </summary>
Active
}
The data model’s state is exposed using a property:
public DataModelState State
{
get
{
VerifyCalledOnUIThread();
return _state;
}
set
{
VerifyCalledOnUIThread();
if (value != _state)
{
_state = value;
OnPropertyChanged("State");
}
}
}
We also implement INotifyPropertyChanged to allow the model to communicate changes in its values.
Since adding\removing event handlers to the PropertyChanged event is a public API exposed by the DataModel, it also requires verification that calls to it are made from the UI thread. We’ll define our own add\remove handlers in order to perform this verification:
protected virtual void OnPropertyChanged(string propertyName)
{
VerifyCalledOnUIThread();
if (_propertyChangedEvent != null)
{
_propertyChangedEvent(this, new PropertyChangedEventArgs(propertyName));
}
}
#region INotifyPropertyChanged Members
public event PropertyChangedEventHandler PropertyChanged
{
add
{
VerifyCalledOnUIThread();
_propertyChangedEvent += value;
}
remove
{
VerifyCalledOnUIThread();
_propertyChangedEvent -= value;
}
}
#endregion
Any property that we’ll add to our data model will call OnPropertyChanged on its setter in order to notify it has changed.
It’s Alive!
One more ability we’d like to add to our DataModel class is the ability to enable\disable it.
As defined earlier, the DataModel encapsulates the logic of fetching data and keeping it “alive” and up to date. To do that, it’ll need to keep some internal timer for updating information or register to some change notification event on its source.
This will keep the DataModel alive and can result in memory leaks, which is why we need some way to turn the DataModel on and off, allowing it to unregister from its data sources when that connection is no longer required:
public bool Enabled
{
get
{
VerifyCalledOnUIThread();
return _isEnabled;
}
set
{
VerifyCalledOnUIThread();
if (value != _isEnabled)
{
_isEnabled = value;
OnPropertyChanged("Enabled");
}
}
}
public void Enable()
{
VerifyCalledOnUIThread();
if (!_isEnabled)
{
this.Enabled = true;
OnEnabled();
}
}
public void Disable()
{
VerifyCalledOnUIThread();
if (_isEnabled)
{
this.Enabled = false;
OnDisabled();
}
}
protected virtual void OnEnabled()
{
}
protected virtual void OnDisabled()
{
}
When binding UI elements to the DataModel we’ll need some mechanism to enable the DataModel when the element is loaded and disable it when the element is unloaded. There’s an elegant way to implement this behavior which we’ll implement in a future post.
That’s it! We’ve got a basic class to derive out data models from. Note that we’re only addressing one-way data binding for the moment. We’ll address a two-way data model (which requires the ability to commit\rollback data etc.) in future post.
On the next post we’ll look into a concrete DataModel implementation for our Stocky application.
You can download the code for this post from here:
Further Reading
- WPF Threading Model - https://msdn2.microsoft.com/en-us/library/ms741870.aspx